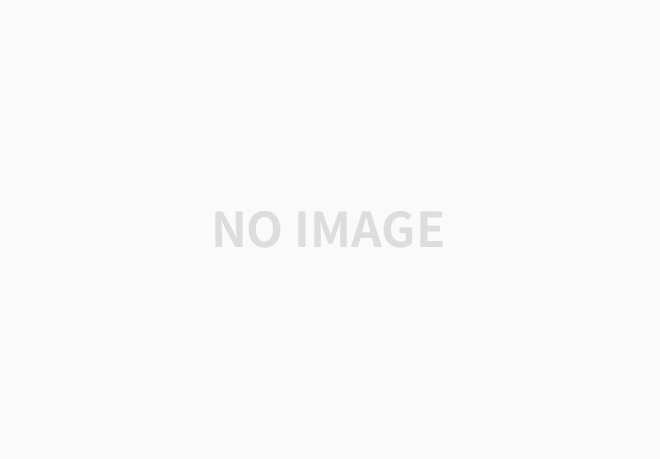
34. JSON(JavaScript Object Notation)
34-1. JSON 정의
34-2. JSON 특징
34-3. JSON 형식
34-4. JSON 유형
34-5. JSON 관련함수
34-6. JSON 교육 전 숙지할 내용
33. async, await
ES7에 추가된 문법.
비동기 처리를 위한 문법 중 하나.
일반적으로 비동기 처리는 callback 함수나 Promise를 사용하지만
async / await는 이를 더욱 간단하게 구현할 수 있도록 도와줌.
async 함수와 await 키워드를 사용하여 구현.
async 함수
항상 Promise를 반환. Promise를 만들고자 하는 함수(=비동기 처리될 전체 함수) 앞에 async를 붙임. return값 필요.
await 키워드
Promise가 처리될 때까지 함수의 실행을 일시 중지하며,
Promise가 처리되면 결과를 반환하고 함수의 실행을 다시 시작. Promise 앞에 await를 붙임.
async function 함수명() { // 해당 함수는 비동기적으로 실행될 것임. ... const A = await 함수a(); // 함수a가 처리될 때까지 다른 코드가 실행되지 않고 대기. const B = await 함수b(); // 함수b가 처리될 때까지 다른 코드가 실행되지 않고 대기. console.log(data); return 값; // Promise 객체에서 resolve()의 값과 동일. } |
예1) Promise3에서의 함수를 async/await를 이용하여 출력하는 코드를 작성.
function getBanana(){ // 1초 후에 '🍌'를 출력하는 함수. return new Promise((resolve)=>{ setTimeout(()=>{ resolve('🍌'); }, 1000); }); } function getApple(){ // 3초 후에 '🍎'를 출력하는 함수. return new Promise((resolve)=>{ setTimeout(()=>{ resolve('🍎'); }, 3000); }); } function getOrange(){ // Error를 출력하는 함수. return Promise.reject(new Error('오렌지는 다팔림')); } // 바나나와 사과를 함께 출력. async function fetchFruits(){ const banana = await getBanana(); // getBanana()가 Promise 함수. const apple = await getApple(); // getApple()이 Promise 함수. return [banana, apple]; // banana와 apple의 출력값을 배열(Array)로 출력. } fetchFruits() .then((fruits)=>console.log(fruits)); // 위의 return 값을 fruits라는 변수에 담아 출력. |
function의 구조
return new Promise( (resolve) => { setTimeout( (resolve) => {출력할내용}, 밀리초) } ) |
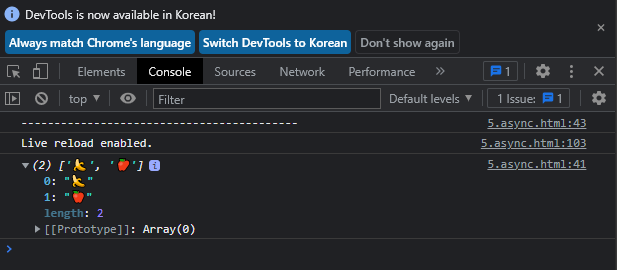
예2) Promise2에서의 함수를 async/await를 이용하여 출력하는 코드를 작성.
function fetchEgg(chicken){ return Promise.resolve(`${chicken} => 🥚`); // chicken을 인자로 받아 Promise가 성공하면 🥚을 반환. } function fryEgg(egg){ return Promise.resolve(`${egg}=>🍳`); // egg를 인자로 받아 Promise가 성공하면 🍳을 반환. } function getChicken(){ return Promise.resolve(`🐤=>🐔`); // Promise가 성공하면 🐤=>🐔을 반환. } async function Chicken_egg(){ // 비동기적으로 실행될 함수. let chicken; // 함수 실행 시 chicken 변수를 초기화함. try { // try-catch문 사용 chicken = await getChicken(); // getChicken()이 처리될 때까지 다른 코드가 실행되지 않고 대기. } catch { chicken = '🐥'; } const egg = await fetchEgg(chicken); // fetchEgg()이 처리될 때까지 다른 코드가 실행되지 않고 대기. return fryEgg(egg); } Chicken_egg() .then(console.log); |
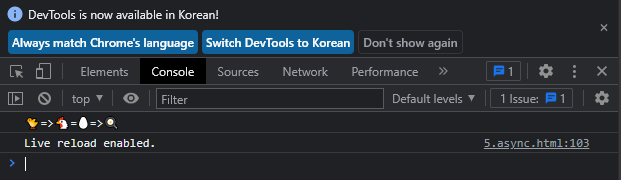
34. JSON(JavaScript Object Notation)
34-1. JSON 정의
텍스트형식으로 데이터의 저장, 전송에 사용되는 경량의 Data 교환 형식.
34-2. JSON 특징
서버와 클라이언트 간의 교류에서 일반적으로 많이 사용.
JavaScript를 이용하여 JSON 형식의 문서를 JavaScript 객체로 변환하기 쉬움.
JavaScript 문법과 굉장히 유사하나, method property가 없는 정렬되지 않은 텍스트 형식.
특정 언어에 종속되지 않으며, 대부분 프로그래밍 언어에서 JSON 포맷의 데이터를 핸들링할 수 있는 라이브러리를 제공.
사람과 기계 모두 이해하기 쉬우며 용량이 작아 XML을 대체하여 데이터 전송등에 많이 사용.
데이터 포맷일뿐, 통신방법이나 프로그래밍 문법이 아님.
34-3. JSON 형식
이름-값 쌍으로 이루어진 데이터 객체를 포함.
데이터는 쉼표(,)로 구분하여 나열.
중괄호({})로 감싸 표현하며 배열은 대괄호([])로 감싸 표현.
{"name":"루시", "age":14, "family":"포메라니안", "weight":3.5} |
34-4. JSON 유형
숫자, 문자열, 불리언, 객체, 배열, null
{ "class": [ {"name":"김사과", "age":20, "hp":"010-1111-1111", "pass":true}, {"name":"반하나", "age":25, "hp":"010-1122-1111", "pass":false}, {"name":"오렌지", "age":30, "hp":"010-1133-1111", "pass":true}, ], [ { }, { }, ] } |
34-5. JSON 관련함수
stringify(object)
객체를 문자열로 변환시키는 JavaScript 함수.
parse(JSON)
문자열을 객체로 변환시키는 JavaScript 함수.
<script> const dog = { name: '루시', age: 14, family: '포메라니안', eat: () => { console.log('잘먹고 잘논다.'); } }; console.log(dog); // object의 형태 const json = JSON.stringify(dog); console.log(json); // {"name":"루시","age":14,"family":"포메라니안"} // json을 객체에서 문자열형태로 변경. const obj = JSON.parse(json); // json을 문자열에서 다시 객체형태로 변경. console.log(obj); // object의 형태 </script> |
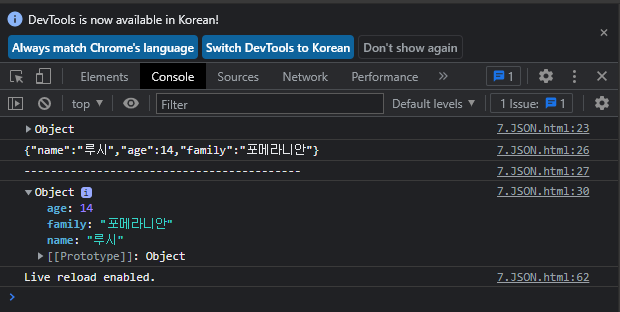
34-6. JSON 교육 전 숙지할 내용
교육 간 사용할 JSON의 예시
https://www.7timer.info/bin/astro.php?lon=113.2&lat=23.1&ac=0&unit=metric&output=json&tzshift=0
https://www.7timer.info/bin/astro.php?ac=0&lat=23.1&lon=113.2&output=json&tzshift=0&unit=metric
www.7timer.info
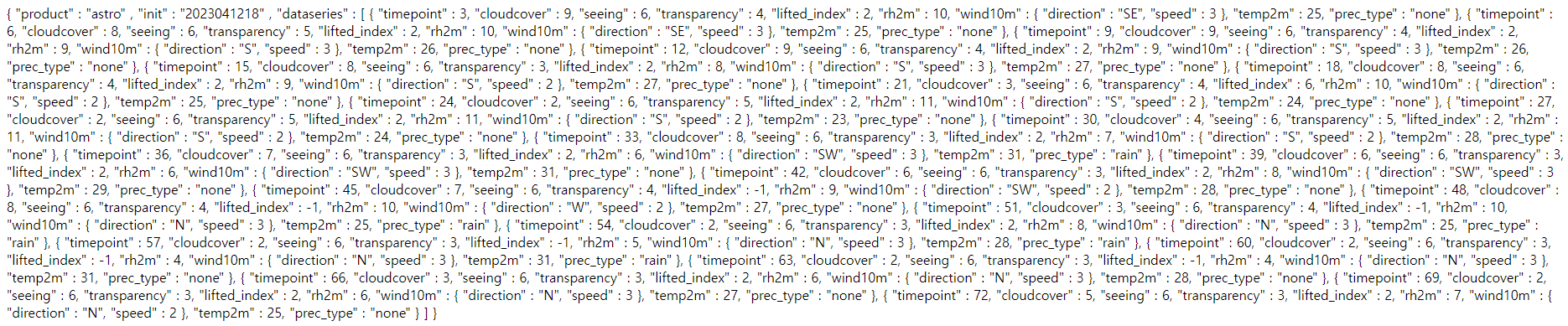
해당 URL(API)를 이용해서 화면을 구성하는데 이용. 프론트엔드 개발자들에 있어서 매우 중요.
JSON의 Error 유무를 검증해주는 웹사이트
(JSONLint: https://jsonlint.com/)
The JSON Validator
JSONLint is the free online validator and json formatter tool for JSON, a lightweight data-interchange format. You can format json, validate json, with a quick and easy copy+paste.
jsonlint.com
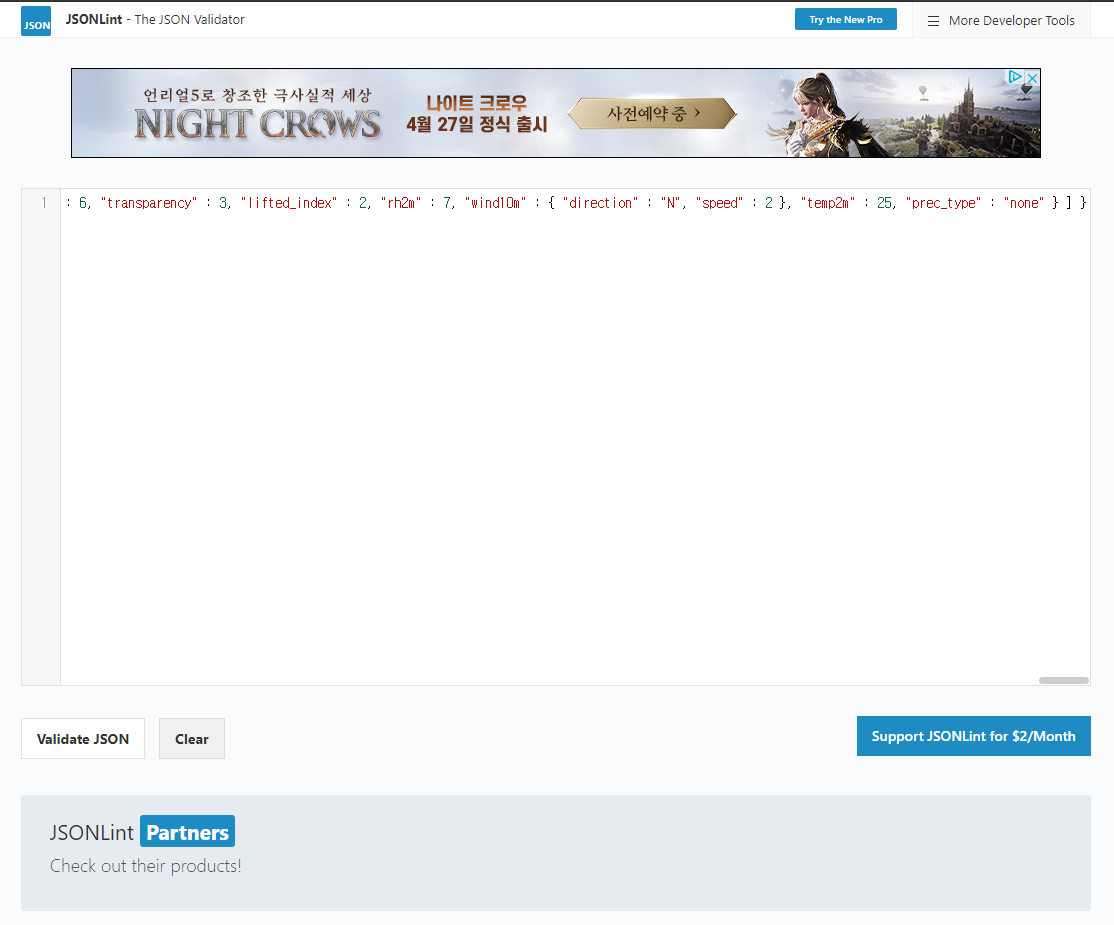
위의 URL을 예시로 복사해서 붙여넣기 후 'Validate JSON' 버튼 클릭
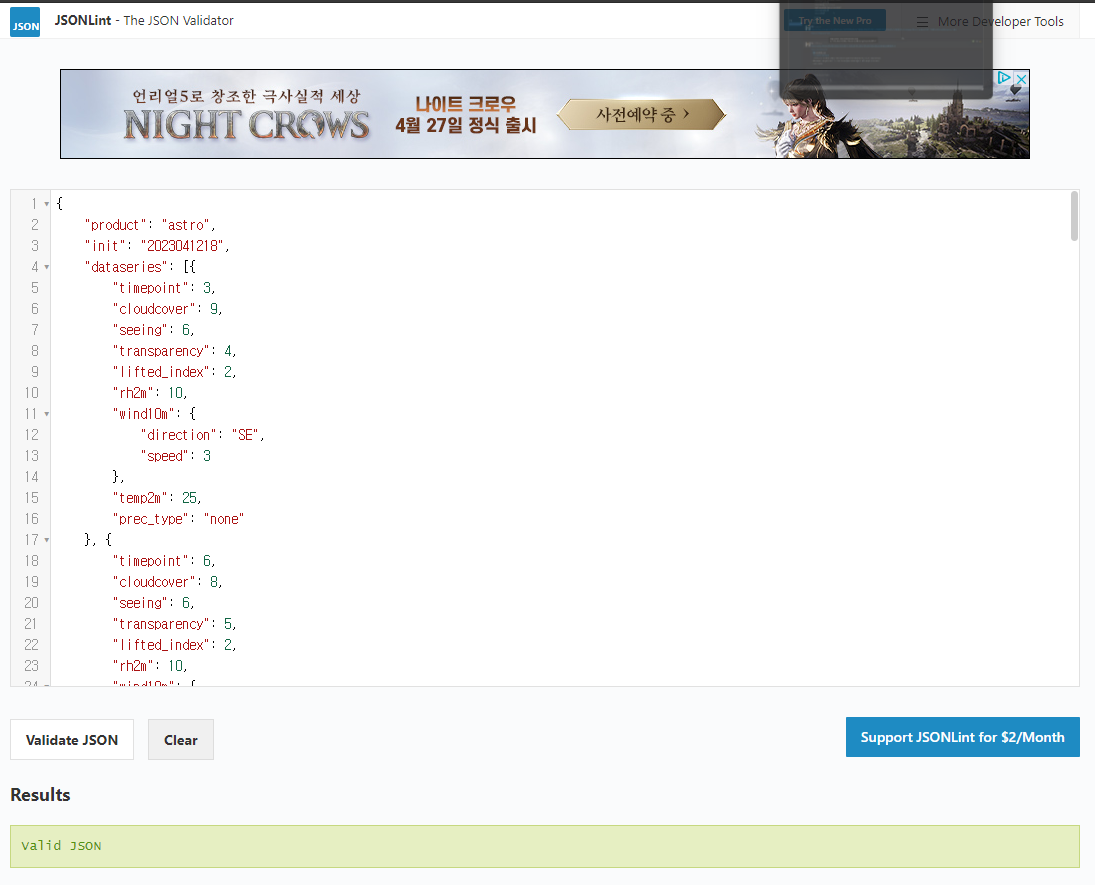
정상적으로 작동할 시, 하단에 연두색 배경으로 'Valid JSON'으로 출력.
JSON의 가시성을 개선해주는 확장 프로그램.
chrome 웹스토어에서 'JSON Formatter'라는 확장프로그램 사용.
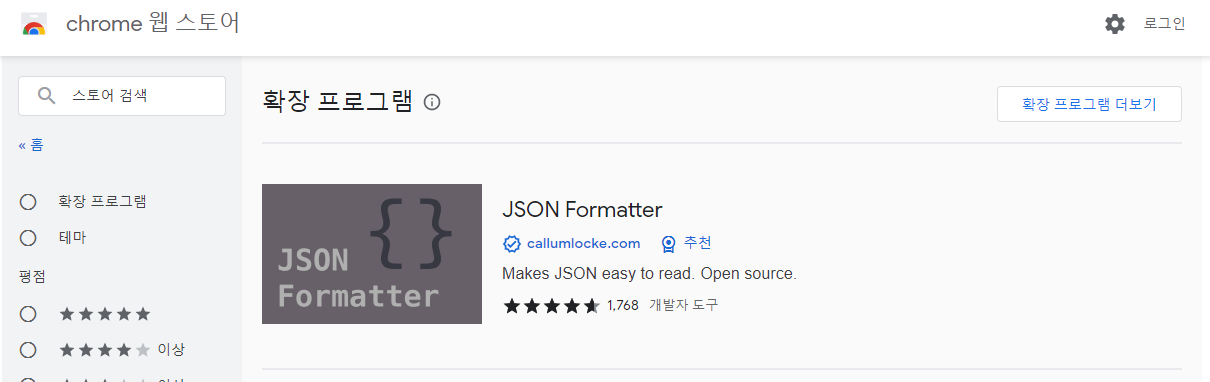
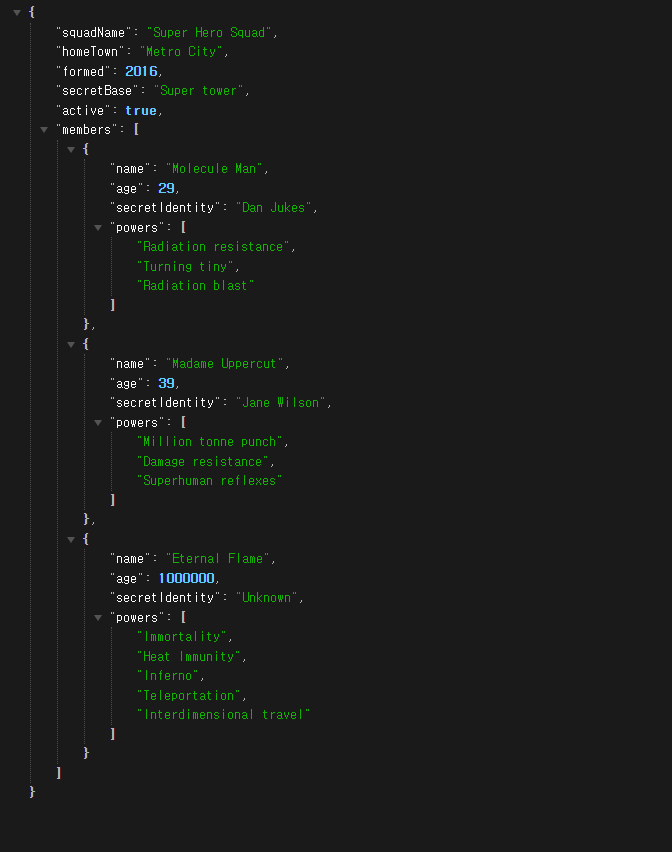
이런식으로 괄호별로 가시성이 좋게 자동으로 변경됨.
35. fetch API
JavaScript에서 제공되는 네트워크 요청을 처리하기 위한 API. 메소드 형식으로 사용.
fetch(url [, options]); |
간결하고 유연한 구조, 보안적인 측면에서도 안전하다는 점이 특징.
Request나 Response와 같은 객체를 이용하여 HTTP 프로토콜을 통해 원격지의 정보를 가져오기 위해 사용.
Promise를 기반으로 동작하며(Promise를 반환), 비동기적으로 작동하므로 callback 함수를 사용하는 것보다
더욱 편리하게 사용할 수 있음.
Ajax와의 비교 Ajax와 Fetch API는 모두 JavaScript에서 비동기적인 네트워크 요청을 처리하기 위한 API. Fetch API가 Ajax보다 더욱 최신의 기술. Ajax는 XMLHttpRequest() 객체를 사용하여 요청을 처리하는 반면, Fetch API는 더욱 간결하고 유연한 구조. Ajax는 오래된 기술이기 때문에, 브라우저 호환성이 좋지 않을 수 있으며, 비동기적인 처리를 위해 복잡한 callback 체인을 만들어야 할 수도 있음. Fetch API는 Promise를 사용하여 비동기적으로 작동하므로 더욱 간편하게 처리할 수 있습니다. Ajax는 기본적으로 Same-Origin Policy를 따르기 때문에, 다른 도메인으로부터의 요청은 제한. Fetch API는 CORS와 같은 보안 문제에 대해 자동으로 처리해주기 때문에 보안적인 측면에서도 안전함. 브라우저 호환성이 좋지 않은 경우에는 Ajax를 사용하는 것이 더욱 적합할 수 있지만, Fetch API는 최신 웹 애플리케이션에서는 더욱 많이 사용되고 있음. |
예) 예시 API(URL)을 이용하여 해당 data를 body에 출력.
<head> <script> function fetchAPI(){ return fetch('https://www.7timer.info/bin/astro.php?lon=113.2&lat=23.1&ac=0&unit=metric&output=json&tzshift=0') .then((response) => response.json()) .then((data) => data); } fetchAPI().then((datas) => { console.log(datas.dataseries); showDatas(datas.dataseries); }); function showDatas(datas){ const ul = document.querySelector('#dataseries'); ul.innerHTML = datas.map((data) => setLiElement(data)).join(''); } function setLiElement(data){ return `<li>cloudcover:${data.cloudcover}, lifted_index:${data.lifted_index}, prec_type:${data.prec_type}, rh2m:${data.rh2m}, seeing:${data.seeing}, temp2m:${data.temp2m}, timepoint:${data.timepoint}, transparency:${data.transparency}</li>`; } </script> </head> <body> <h2>fetch</h2> <ul id="dataseries"> </ul> </body> |
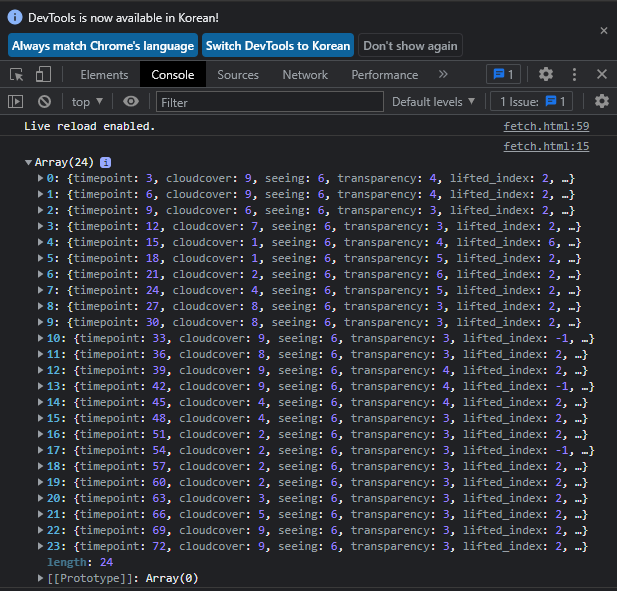
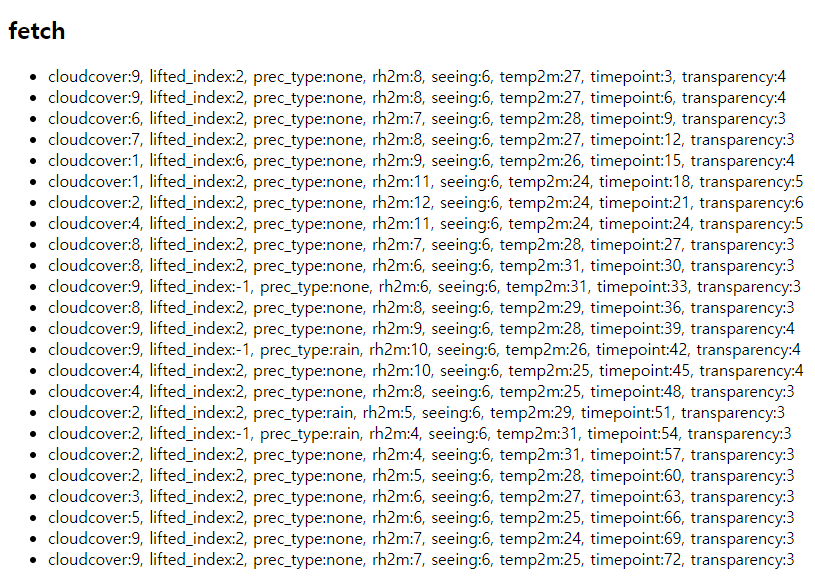
TIP
font를 무료로 가져오는 웹사이트
fontawesome: https://fontawesome.com/
Font Awesome
The internet's icon library + toolkit. Used by millions of designers, devs, & content creators. Open-source. Always free. Always awesome.
fontawesome.com